李宏毅机器学习课程(1)Pytorch Tutorial
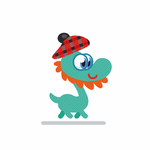
Pytorch作为深度学习常用模块库,里面包含了很多深度学习相关的操作和模块,底层由C++实现,使用张量进行计算,可使用GPU加速。
Pytorch Documentation
参考网址:https://pytorch.org/docs/stable/
常用的模块有:
- torch.nn -> neural nework
- torch.optim -> optimization algorithms
- torch.utils.data -> dataset, dataloader
Pytorch Documentation Example
Function inputs and outputs
Data type and explanation of each input
Some functions behave differently with different inputs
在PyTorch中,参数(parameters)和关键字参数(keyword arguments)是两种不同的概念。
参数是模型中的可学习参数,例如线性层中的权重。它们通常被定义为模型的nn.Module
的成员变量,并且可以通过调用nn.Module
的成员函数parameters()
返回。
关键字参数是函数的额外参数,它们是可选的。它们可以通过向函数提供以参数名称为关键字的额外参数的形式来提供,例如:nn.Linear(in_features, out_features, bias=True)
。
三种不同输入对应的torch.max
Common Errors
Tensor on Different Device to Model
1 |
|
Tensor for * is on CPU, but expected them to be on GPU
=> send the tensor to GPU
1 |
|
Mismatched Dimensions
1 |
|
The size of tensor a (5) must match the size of tensor b (4) at non-singleton dimension 1
=> the shape of a tensor is incorrect, use transpose, squeeze, unsqueeze to align the dimensions
1 |
|
Cuda Out of Memory
1 |
|
CUDA out of memory. Tried to allocate 350.00 MiB (GPU 0; 14.76 GiB total capacity; 11.94 GiB already allocated; 123.75 MiB free; 13.71 GiB reserved in total by PyTorch)
=> The batch size of data is too large to fit in the GPU. Reduce the batch size.
Mismatched Tensor Type
1 |
|
expected scalar type Long but found Float
=> labels must be long tensors, cast it to type “Long” to fix this issue
1 |
|
- Title: 李宏毅机器学习课程(1)Pytorch Tutorial
- Author: 茴香豆
- Created at : 2023-02-15 07:24:12
- Updated at : 2023-02-15 08:26:06
- Link: https://hxiangdou.github.io/2023/02/15/ML-LHY-1/
- License: This work is licensed under CC BY-NC-SA 4.0.