动手学习深度学习(6)模型选择与欠拟合和过拟合
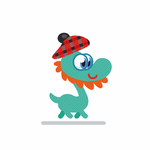
我们如何才能确定模型是真正发现了一种泛化的模式, 而不是简单地记住了数据呢? 我们的目标是发现某些模式, 这些模式捕捉到了我们训练集潜在总体的规律。 如果成功做到了这点,即使是对以前从未遇到过的个体, 模型也可以成功地评估风险。 如何发现可以泛化的模式是机器学习的根本问题。
误差:
- 训练误差(training error):模型在训练数据集上计算得到的误差
- 泛化误差(generalization error):模型应用在同样从原始样本的分布中抽取的无限多数据样本时模型误差的期望。简单来说,是模型在新数据上的误差。
数据集:(验证数据集一定不要和测试数据集混合在一起!)
- 验证数据集:一个用来评估模型好坏的数据集
- 测试数据集:只用一次的数据集
通常没有足够数据进行使用,所以常采用K折交叉验证,一般取5或10
过拟合和欠拟合
数据简单 | 数据复杂 | |
---|---|---|
模型容量低 | 正常 | 欠拟合 |
模型容量高 | 过拟合 | 正常 |
模型容量:拟合各种函数的能力
- 低容量的模型难以拟合训练数据
- 高容量的模型可以记住所有的训练数据
实际中一般靠观察训练误差和验证误差的差值,过小,欠拟合,过大,过拟合。
一些正则化模型的技术
权重衰退(weight-decay)
通过限制参数值的选择范围来控制模型容量,达到避免过拟合的效果
MATHJAX-SSR-23
- 通常不限制偏移b
- 小的 意味着更强的正则项
可以使用均方范数作为柔性限制。对于每个 MATHJAX-SSR-24,都可以找到 使得上面的目标函数等价于下面的公式
MATHJAX-SSR-25
超参数 控制了正则项的重要程度
- = 0 :无作用
- -> , 最优解 -> 0
从零实现
1 |
|
简洁实现
1 |
|
丢弃法(dropout)
丢弃法将一些输出项随机置0来控制模型复杂度,只用在训练过程中,预测过程会被去掉。
在数据中加入噪音,等价于一个正则。丢弃法是在全连接层间(后)加入噪音。通常将丢弃法作用在隐藏全连接层的输出上,即下一层的输入是上一层输出的进行dropout后的数据。
MATHJAX-SSR-26
根据此模型的设计,其期望值保持不变,即 。
从零开始实现
1 |
|
简洁实现
1 |
|
- Title: 动手学习深度学习(6)模型选择与欠拟合和过拟合
- Author: 茴香豆
- Created at : 2022-10-28 16:17:57
- Updated at : 2022-10-29 10:13:39
- Link: https://hxiangdou.github.io/2022/10/28/DL_6/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments