Python学习之路(14)使用API【项目2 数据可视化】
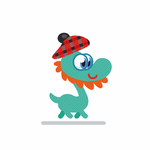
在本章中,你将学习如何编写一个独立的程序,并对其获取的数据进行可视化。
1.使用Web API
本章的可视化将基于来自GitHub的信息,这是一个让程序员能够协作开发 项目的网站。我们将使用GitHub的API来请求有关该网站中Python项目的信 息,然后使用Pygal
生成交互式可视化,以呈现这些项目的受欢迎程度。
1 |
|
1 |
|
仓库相关信息
1 |
|
2.使用Pygal可视化仓库
创建条形图表示项目获得了多少颗星星。
1 |
|
3.Hacker News API
下面的调用返回本书编写时最热门的文章的信息
1 |
|
响应是一个字典,包含ID为9884165的文章的信息
1 |
|
执行一个API调用,返回Hacker News上当前热门文章的ID,再查看 每篇排名靠前的文章
1 |
|
- Title: Python学习之路(14)使用API【项目2 数据可视化】
- Author: 茴香豆
- Created at : 2022-10-11 10:28:00
- Updated at : 2022-10-11 16:07:57
- Link: https://hxiangdou.github.io/2022/10/11/Python_14/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments