动手学习深度学习(2)简单的数学知识
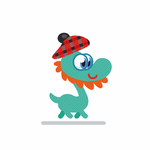
本节主要讲解线性代数,按特定轴求和,矩阵计算和自动求导相关内容
1. 线性代数
矩阵的特征值和特征向量。
矩阵的转置。
2. 矩阵计算
常用函数
1 |
|
按特定轴求和
举例比较好理解,例如,对于2x3x4的矩阵:
- 若axis=0,求sum后矩阵变为二维矩阵,大小为3x4
- 若axis=1,求sum后矩阵变为二维矩阵,大小为2x4
- 若axis=2,求sum后矩阵变为二维矩阵,大小为2x3
- 若axis=[0, 1],求sum后矩阵变为一维矩阵,长度为4
- 若axis=[0, 2],求sum后矩阵变为一维矩阵,长度为3
- 若axis=[1, 2],求sum后矩阵变为一维矩阵,长度为2
- 若axis=[0, 1, 2],求sum后矩阵变为一个数
1 |
|
计算总和或均值时保持轴数不变
保持求和后的矩阵与求和前的矩阵形状相同。
1 |
|
应用:使得我们可以通过广播将A
除以sum_A
1 |
|
3. 自动求导
矩阵求导的本质与分子布局、分母布局的本质(矩阵求导——本质篇)
基本向量求导运算:
矩阵求导运算后的形状:
计算图:
- 将代码分解为操作子
- 将计算表示成一个无环图
自动求导实现
假设我们想对函数 关于列向量 求导。在我们计算 关于 的梯度之前,我们需要一个地方来存储梯度。
1 |
|
调用反向传播函数来自动计算 关于 每个分量的梯度。
1 |
|
现在让我们计算 的另一个函数。
1 |
|
深度学习中,我们的目的不是计算微分矩阵,而是批量中每个样本单独计算的偏导数之和。
1 |
|
将某些计算移动到记录的计算图之外。
1 |
|
- Title: 动手学习深度学习(2)简单的数学知识
- Author: 茴香豆
- Created at : 2022-09-24 19:03:10
- Updated at : 2023-02-23 11:44:24
- Link: https://hxiangdou.github.io/2022/09/24/DL_2/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments